类图查看器
说明
类图查看器使您能够创建显示类实现细节和层次结构的图。您可以使用此 App 来探查类结构体并与其他人共享类图。这些图可以包括有关以下各项的详细信息:
类的内部结构,包括属性和方法及其访问属性
类的层次结构,包括命名空间,显示继承关系
您可以使用图形界面或由 matlab.diagram.ClassViewer
定义的命令行 API 来创建类图。
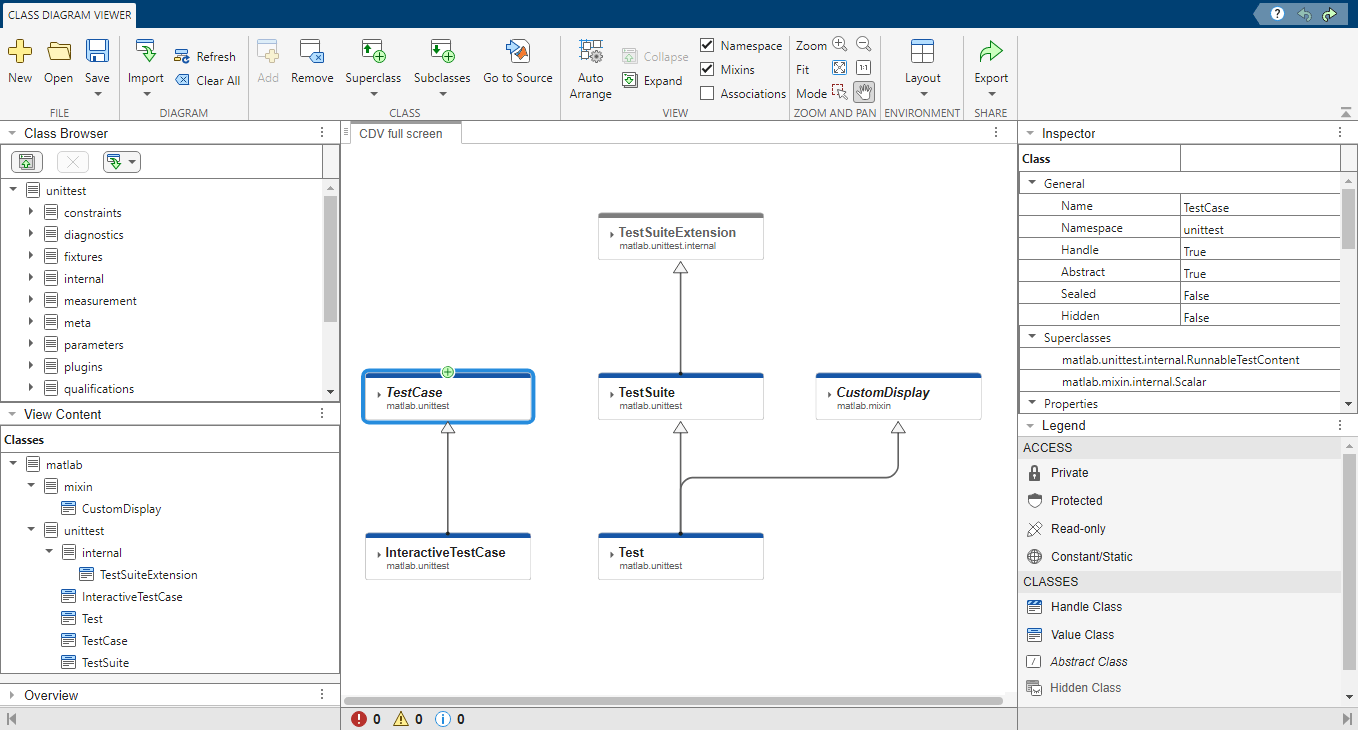
打开 类图查看器
MATLAB® 工具条:在 App 选项卡上的 MATLAB 下,点击 App 图标。
MATLAB 命令提示符:输入
matlab.diagram.ClassViewer
。
示例
创建和保存简单类图
打开类图查看器。在类浏览器窗格中,展开“导入类、命名空间或文件夹”按钮 ,然后选择导入命名空间。输入
matlab.unittest
作为命名空间,然后点击确定。unittest
所在的文件夹将显示在类浏览器中。
展开 unittest
文件夹并选择 InteractiveTestCase
类。点击工具条中的添加按钮,以将一个代表该类的卡片添加到类图查看器画布中。使用相同的方法添加 TestCase
类。由于 TestCase
是 InteractiveTestCase
的超类,类图查看器会自动绘制一个从 InteractiveTestCase
到 TestCase
的箭头来显示这种关系。
类卡顶部的斜纹蓝色边框表示这两个类均为句柄类。图例窗格说明画布上的图形效果和图标表示的意义。
您也可以将项目从类浏览器直接拖到画布上。将 Test
类拖到查看器中。卡顶部的 表示
Test
有可查看的超类。
点击 可将该超类添加到图中。从
Test
指向 TestSuite
的箭头表示 TestSuite
是 Test
的超类。
除了通过拖放在查看器中移动类之外,您还可以使用工具条的缩放和平移部分中的选项来自定义视图,包括:
放大和缩小
使图适应当前屏幕大小
平移
在工具条的视图部分中,选中 Mixins 复选框。此操作不会自动将 mixin 类添加到图中,但随后当您执行任何添加超类的操作时,查看器会将它们添加到显示中。
要添加 Test
的所有超类,请右键点击 Test
类卡,然后选择添加所有超类。现在,CustomDisplay
mixin 显示为 Test
的超类,TestSuiteExtension
显示为 TestSuite
的超类。CustomDisplay
的名称为斜体,表示它是抽象类。
添加超类和子类时有两种选择:
添加超类:添加给定类直接从其继承的超类。
添加所有超类:添加给定类直接或间接从其继承的所有超类。在上面的示例中,
Test
间接从TestSuiteExpansion
继承。添加子类:添加直接从给定类继承的已知子类。
添加所有子类:添加所有直接或间接从给定类继承的已知子类。
注意
MATLAB 可能无法识别给定类的所有现有子类。在这种情况下,请使用类浏览器手动添加任何其他子类。
除了显示类层次结构,您还可以探索类定义本身。通过点击卡中类名称旁边的箭头,展开 Test
类卡。类卡会展开以显示由类定义的属性和方法。从超类继承的属性和方法不会出现在子类卡中。
属性和方法名称旁边的图标标识属性和方法的访问权限级别。例如,锁图标显示 ExternalFixtures
是私有属性。
选择类卡上的属性或方法还会在检查器窗格中显示访问权限和其他信息。
要查看一个或多个类的源代码,请选择类卡,然后点击工具条中的转至源。源代码文件在 MATLAB 编辑器中打开。
要保存或共享您的图,请使用以下两个选项之一:
点击导出以将图保存为静态图像。
选择保存 > 另存为以创建一个 MLDATX 文件,该文件可以在类图查看器实例中重新打开和进行编辑。
向图中添加关联
自 R2024a 起
类图查看器可以显示类之间的关联。当使用类验证将一个类属性的类型显式定义为另一个类时,类图查看器可以显示这种关联。
将这两个类保存到您的 MATLAB 路径。
classdef ClassA properties Property1 ClassB PropertySelf ClassA end end
classdef ClassB properties Property1 double end end
打开类图查看器。在类浏览器窗格中,展开“导入类、命名空间或文件夹”按钮 ,然后选择导入类。输入
ClassA
,然后点击确定。对 ClassB
重复此步骤。这两个类都出现在类浏览器中。
将 ClassA
和 ClassB
从类浏览器拖到画布上。通过点击卡中类名称旁边的箭头,展开 ClassA
类卡。类卡会展开以显示由类定义的属性和方法。
在工具条的视图部分中,选中关联复选框。此操作将箭头添加到图中,用于任何可识别的关联。在这种情况下:
ClassA
的Property1
属于ClassB
类型,因此箭头将Property1
连接到ClassB
的类卡。ClassA
的PropertySelf
属于ClassA
类型,因此箭头将该属性连接回ClassA
的主卡。
使用关联复选框可以打开和关闭箭头。即使类卡折叠,箭头也会出现。
当使用类验证定义属性时,类图查看器只能识别关联。以这种方式定义时,属性的类将显示在类卡上。有关详细信息,请参阅Property Class Validation。此外,打开关联只会显示当前图中的类之间的连接。例如,如果 ClassB
不在图中,App 不会自动添加它来显示与 ClassA
的 Property1
的关联。
编程用途
matlab.diagram.ClassViewer
matlab.diagram.ClassViewer
打开一个未加载类的类图查看器实例。
matlab.diagram.ClassViewer(Name=Value
)
Name=Value
)matlab.diagram.ClassViewer(
将指定的类添加到类浏览器窗格和画布中。有效的名称-值参量包括:Name=Value
)
Classes
:类名称指定为字符串或对象名称Folders
:文件夹名称指定为字符串Namespaces
:命名空间名称指定为字符串
有关其他名称-值参量,请参阅 matlab.diagram.ClassViewer
。将参量指定为 Name1=Value1
,...,NameN=ValueN
,其中 Name
是参量名称,Value
是对应的值。
提示
类图查看器是
matlab.diagram.ClassViewer
类的实例。使用类构造函数一次打开多个查看器。如果
出现在两个类之间的箭头上而不是在类卡本身上,则两个连接的类之间的层次结构中还有其他类。点击
可添加层次结构中该部分的所有可查看的类。
如果在使用类图查看器期间您更改了类代码,可以通过点击刷新来自动更新图。如果类文件被删除或对 App 不再可用,App 会指示类未同步,但类卡本身不会从图中删除。
版本历史记录
在 R2021a 中推出R2024a: 显示关联
类图查看器现在可以识别和显示类之间的关联。当使用类验证将一个类属性的类型显式定义为另一个类时,类图查看器可以通过用箭头将该属性连接到其类型的类卡来显示这种关联。
R2024a: 包现在称为命名空间
MATLAB 现在将包称为命名空间。该 App 及其命令行界面 (matlab.diagram.ClassViewer
) 已更新以反映此术语变更。其行为保持不变。
R2023b: 用于添加子类的新选项
现在,在添加子类时有两个新的选项。可以右键点击类卡或使用工具条中的图标来访问这些选项。
添加子类:添加直接从给定类继承的已知子类。
添加所有子类:添加所有直接或间接从给定类继承的已知子类。
MATLAB 可能无法识别给定类的所有现有子类。在这种情况下,请使用类浏览器手动添加任何其他子类。
MATLAB 命令
您点击的链接对应于以下 MATLAB 命令:
请在 MATLAB 命令行窗口中直接输入以执行命令。Web 浏览器不支持 MATLAB 命令。
Select a Web Site
Choose a web site to get translated content where available and see local events and offers. Based on your location, we recommend that you select: .
You can also select a web site from the following list:
How to Get Best Site Performance
Select the China site (in Chinese or English) for best site performance. Other MathWorks country sites are not optimized for visits from your location.
Americas
- América Latina (Español)
- Canada (English)
- United States (English)
Europe
- Belgium (English)
- Denmark (English)
- Deutschland (Deutsch)
- España (Español)
- Finland (English)
- France (Français)
- Ireland (English)
- Italia (Italiano)
- Luxembourg (English)
- Netherlands (English)
- Norway (English)
- Österreich (Deutsch)
- Portugal (English)
- Sweden (English)
- Switzerland
- United Kingdom (English)