主要内容
搜索
A key aspect to masting MATLAB Graphics is getting a hang of the MATLAB Graphics Object Hierarchy which is essentially the structure of MATLAB figures that is used in the rendering pipeline. The base object is the Graphics Root (see groot) which contains the Figure. The Figure contains Axes or other containers such as a Tiled Chart Layout (see tiledlayout). Then these Axes can contain graphics primatives (the objects that contain data and get rendered) such as Lines or Patches.
Every graphics object has two important properties, the "Parent" and "Children" properties which can be used to access other objects in the tree. This can be very useful when trying to customize a pre-built chart (such as adding grid lines to both axes in an eye diagram chart) or when trying to access the axes of a non-current figure via a primative (so "gca" doesn't help out).
One last Tip and Trick with this is that you can declare graphics primatives without putting them on or creating an Axes by setting the first input argument to "gobjects(0)" which is an empty array of placeholder graphics objects. Then, when you have an Axes to plot the primitive on and are ready to render it, you can set the "Parent" of the object to your new Axes.
For Example:
l = line(gobjects(0), 1:10, 1:10);
...
...
...
l.Parent = gca;
Practicing navigating and exploring this tree will help propel your understanding of plotting in MATLAB.
We're thrilled to announce the roll-out of some new features that are going to supercharge your Playground experience! Here's what's new:
Copy/Download code from the script area
You can now effortlessly Copy/Download code from the script area with just a single click. Copy code or Download your script directly as .m files and keep your work organized and portable.We hope this will allow you to effortlessly transfer your work from Playground to MATLAB Desktop/Online.
Run Code directly from the Chat panel
Execute code snippets from the chat section with a single click. This new affordance means saving a step since you no longer have to insert code and then hit run from the toolstrip to execute instead just hit run in the chat panel to see the output immediately in the script area
Enhanced visual Experience
Customize your Playground workspace by expanding or collapsing the chat and script sections. Focus on what matters most to you, whether it's AI chat or working on your script.
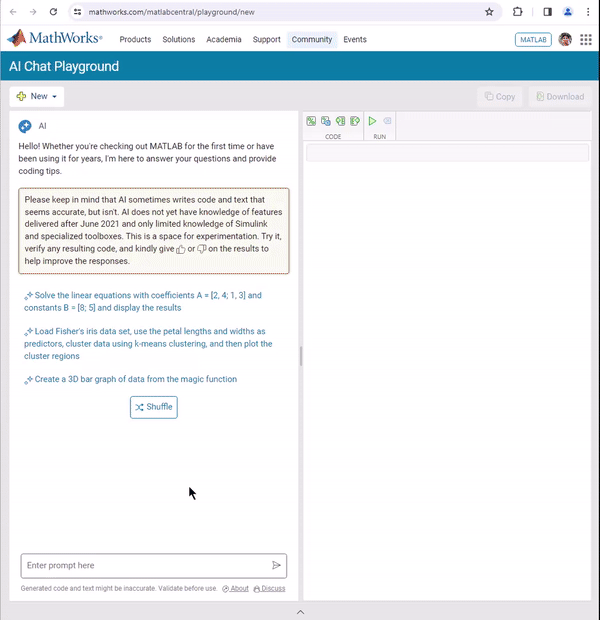
We hope you will love these updates. Try them out and let us know your feedback.
When I want to understand a problem, I'll often use different sources. I'll read different textbooks, blog posts, research papers and ask the same question to different people. The differences in the solutions are almost always illuminating.
I feel the same way about AIs. Sometimes, I don't want to ask *THE* AI...I want to ask a bunch of them. They'll have different strengths and weaknesses..different personalities if you want to think of it that way.
I've been playing with the AI chat arena and there really is a lot of difference between the answers returned by different models. https://lmarena.ai/?arena
I think it would be great if the MATLAB Chat playgroundwere to allow the user to change which AI they were talking with.
What does everyone else think?
how can i use this AI?
I have been finding the AI Chat Playground very useful for daily MATLAB use. In particular it has been very useful for me in basically replacing or supplementing dives into MATLAB documentation. The documentation for MATLAB is in my experience uniformly excellent and thorough but it is sometimes lengthy and hard to parse and the AI Chat is a great one stop shop for many questions I have. However, I would find it very useful if the AI Chat could answer my queries and then also supply a link directly to the documentation. E.g. a box at the bottom of the answer that is basically
"Here is the documentation on the functions AI Chat referred to in this response"
could be neat.
I recently wrote about the new ODE solution framework in MATLAB over the The MATLAB Blog The new solution framework for Ordinary Differential Equations (ODEs) in MATLAB R2023b » The MATLAB Blog - MATLAB & Simulink (mathworks.com)
This was a very popular post at the time - many thousands of views. Clearly everyone cares about ODEs in MATLAB.
This made me wonder. If you could wave a magic wand, what ODE functionality would you have next and why?
Over at Reddit, a MATLAB user asked about when to use a script vs. a live script. How would you answer this?
Hi
I am using simulink for the frequency response analysis of the three phase induction motor stator winding.
The problem is that i can't optimise the pramaeter values manually, for this i have to use genetic algrothem. But iam stucked how to use genetic algorithum to optimise my circuit paramter values like RLC. Any guidence will be highly appreciated.
Starting with MATLAB can be daunting, but the right resources make all the difference. In my experience, the combination of MATLAB Onramp and Cody offers an engaging start.
MATLAB Onramp introduces you to MATLAB's basic features and workflows. Then practice your coding skill on Cody. Challenge yourself to solve 1 basic problem every day for a month! This consistent practice can significantly enhance your proficiency.
What other resources have helped you on your MATLAB journey? Share your recommendations and let's create a comprehensive learning path for beginners!
I am a beginner of deep learning, and meet with some problems in learning the MATLAB example "Denoise Signals with Adversarial Learning Denoiser Model", hope very much to get help!
1. visualizaition of the features
It is my understanding that the encoded representation of the autoencoder is the features of the original signal. However in this example, the output dimension of the encoder is 64xSignalLength. Does it mean that every sample point of the signal has 64 features?
2. usage of the residual blocks
The encoder-decoder model uses residual blocks (which contribute to reconstructing the denoised signal from the latent space, ). However, only the encoder output is connected to the discriminator. Doesn't it cause the prolem that most features will be learned by the residual blocks, and only a few features that could confuse the discriminator will be learned by the encoder and sent to the discriminator?

I would tell myself to understand vectorization. MATLAB is designed for operating on whole arrays and matrices at once. This is often more efficient than using loops.
Is there a reason for TMW not to invest in 3D polyshapes? Is the mathematical complexity of having all the same operations in 3D (union, intersection, subtract,...) prohibitive?
I have been developing a neural net to extract a set of generative parameters from an image of a 2-D NMR spectrum. I use a pair of convolution layers each followed by a fullyconnected layer; the pair are joined by an addtion layer and that fed to a regression layer. This trains fine, but answers are sub-optimal. I woudl like to add a fully connected layer between the addtion layer and regression, but training using default training scripts simply won't converge. Any suggestions? Maybe I can start with the pre-trained weights for the convolution layers, but I don't know how to do this.
JHP
This is not a question, it is my attempt at complying with the request for thumbs up/down voting. I vote thumbs up, for having AI.....
I am not sure if specific AI errors are to be reported. Other messages I just read from others here and the AI Chat itself clearly state that errors abound.
My AI request was: "Plot 300 points of field 2"
AI Chat gave me, in part:
data = thingSpeakRead(channelID, 'Fields', 2, 'NumPoints', 300, 'ReadKey', readAPIKey);
% Extract the field values
field1Values = data.Field1;
% Plot the data
plot(field1Values);
The AI code failed due to "Dot indexing is not supported for variables of this type"
So, I corrected the code thus to get the correct plot:
data = thingSpeakRead(channelID, 'Fields', 2, 'NumPoints', 300, 'ReadKey', readAPIKey);
% Extract the field values
%field1Values = data.Field1;
% Plot the data
plot(data);
I see great promise in AI Chat.
Opie
Explore all the capabilities for Modeling Dynamic Systems while keeping them handy with this Cheat Sheet - Download Now.

Quick answer: Add set(hS,'Color',[0 0.4470 0.7410]) to code line 329 (R2023b).
Explanation: Function corrplot uses functions plotmatrix and lsline. In lsline get(hh(k),'Color') is called in for cycle for each line and scatter object in axes. Inside the corrplot it is also called for all axes, which is slow. However, when you first set the color to any given value, internal optimization makes it much faster. I chose [0 0.4470 0.7410], because it is a default color for plotmatrix and corrplot and this setting doesn't change a behavior of corrplot.
Suggestion for a better solution: Add the line of code set(hS,'Color',[0 0.4470 0.7410]) to the function plotmatrix. This will make not only corrplot faster, but also any other possible combinations of plotmatrix and get functions called like this:
h = plotmatrix(A);
% set(h,'Color',[0 0.4470 0.7410])
for k = 1:length(h(:))
get(h(k),'Color');
end
Write a matlab script that will print the odd numbers, 1 through 20, in reverse.
I cannot figure out how to do this correctly, please help.