adapt histogram equalization- clahe
4 次查看(过去 30 天)
显示 更早的评论
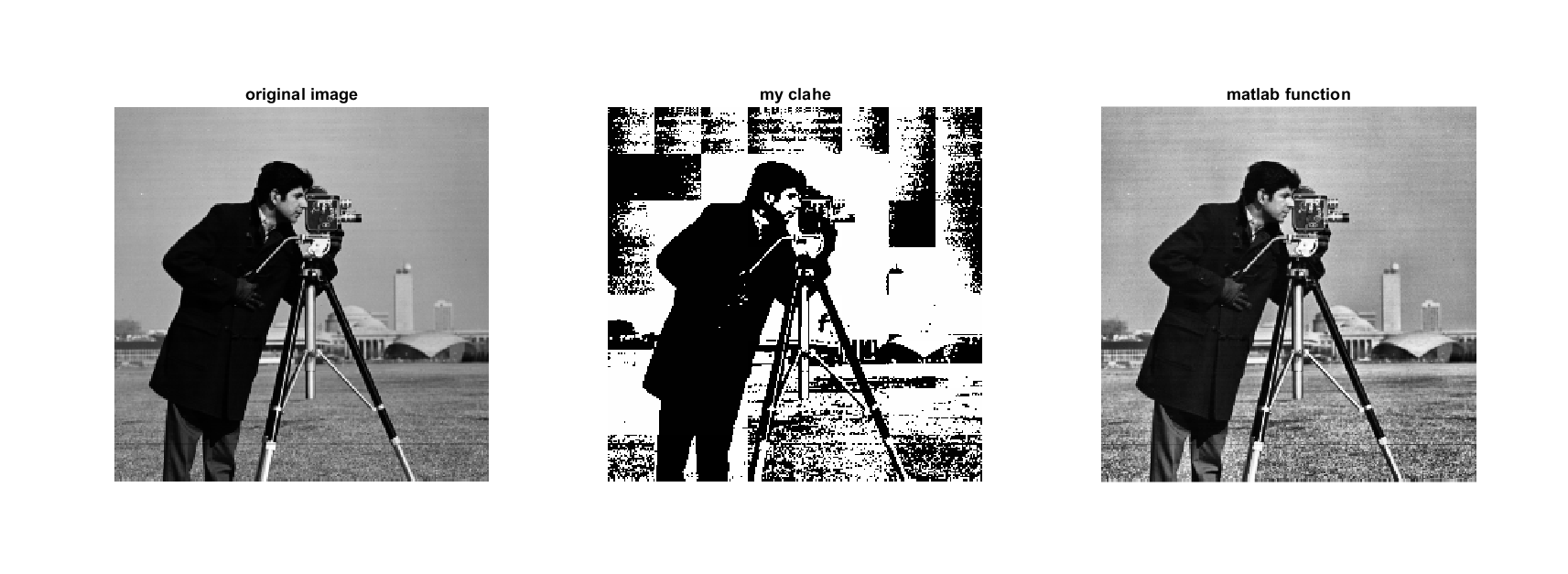
I have a problem with the final interpolation I think. the connection between the tiles is very visible and each tile has a different colloring.
Any one have an idea how i can make this better?
1 个评论
DGM
2024-7-23
编辑:DGM
2024-7-23
It's hard to diagnose unseen code, but based on the extreme contrast in the output image, I'd suspect that you're operating at some point on improperly-scaled data (e.g. uint8-scale float instead of unit-scale float). If that's the case, it's not clear whether the output could be fixed by rescaling after the fact, or if it's already suffered truncation at some point.
That's just a guess.
回答(1 个)
Aastha
2024-9-27
As I understand, you want to implement adaptive histogram equalization with contrast limiting while also addressing the artifacts in your method.
These artifacts, known as tiling artifacts, occur when different tiles display varying colours. To mitigate this, you can use overlapping tiles along with a blending mechanism.
In the code snippet attached below, you can set the stride parameter to be less than half the size parameter, which will ensure the windows overlap. Additionally, you can apply a Gaussian blending mechanism to smoothly transition the colours between tiles.
You can do this using the following steps:
1.First, you can start by reading an input image using the “imread” function and checking whether the image is RGB. If it is, you can convert it to grayscale; otherwise, you can use the image as it is. The code snippet below illustrates this:
input_image = imread('cameraman.tif');
if size(input_image, 3) == 3
grayscale_image = rgb2gray(input_image);
else
grayscale_image = input_image;
end
You may refer to the documentation of “imread” function for more information. Here is the link to it:
2.Next, you can define the sliding window size and stride for the adaptive histogram equalization process. You can also specify a standard deviation value for the Gaussian blending mask, which controls how smooth the blending will be. You may refer to the code snippet below to do this:
window_size = [64, 64];
stride = [8, 8];
sigma = 10;
3.To create a Gaussian blending mask, you can use a 2D Gaussian distribution over a grid that matches the size of the sliding window. This mask will help you smoothly blend the histogram-equalized image sections.
[X, Y] = meshgrid(linspace(-1, 1, window_size(2)), linspace(-1, 1, window_size(1)));
G = exp(-(X.^2 + Y.^2) / (2 * sigma^2));
For more information on “meshgrid” function, you may refer to the documentation whose link is mentioned below:
4.You can then apply the adaptive histogram equalization by moving the window across the image with the specified stride. For each window, you can perform histogram equalization and blend the result into the output image
using the Gaussian mask. You will also update the weight map to accumulate blending weights.
for i = 1:stride(1):rows-window_size(1)+1
for j = 1:stride(2):cols-window_size(2)+1
window = grayscale_image(i:i+window_size(1)-1, j:j+window_size(2)-1);
equalized_window = histeq(window);
equalized_image(i:i+window_size(1)-1, j:j+window_size(2)-1) = ...
equalized_image(i:i+window_size(1)-1, j:j+window_size(2)-1) + ...
double(equalized_window) .* G;
weight_map(i:i+window_size(1)-1, j:j+window_size(2)-1) = ...
weight_map(i:i+window_size(1)-1, j:j+window_size(2)-1) + G;
end
end
5. Finally, you can normalize the resulting equalized image by dividing it by the accumulated weight map.
equalized_image = uint8(equalized_image ./ weight_map);
I hope this helps!
0 个评论
另请参阅
Community Treasure Hunt
Find the treasures in MATLAB Central and discover how the community can help you!
Start Hunting!