Results for
Check out this pick of the week from Emma Gau. Emma's submission is featured on the Live Script gallery. Check out the blog post to see why Owen picked it.

Check out this pick of the week from Will. Steve's progressbar submission has been around since 2005 and still runs perfectly. Check out the blog post to see why Will picked it.
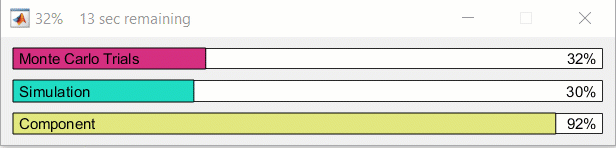
We are excited to announce that Adam Danz has accepted our invitation and now is a member of the Community Advisory Board (CAB)!
Adam has been a rising star in Answers, obtaining 4500+ reputation points in the past year! Furthermore, he has contributed high-quality files to File Exchange, with an average rating of 4.8. Adam also demonstrates good communication skills and the ability to work with others. Those characteristics are what we expect to see from a CAB advisor. You can learn more about him and CAB on the CAB page .
On behalf of all the community team, we would like to extend our warmest welcome to Adam!
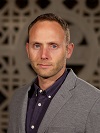
Check out Sean's blog posts ( Intro , Authoring ) on Spider Plots. He's using spider_plot by Moses to create beautiful plots like this.

The following is a list of updates and new features for MATLAB Central, including MATLAB Answers, File Exchange, Blogs, and Cody.
New Features
Community highlights channel released - See this post for more details.
Answers comment UI change - A minor improvement to the user interface when adding comments to questions and answers in MATLAB Answers. This change should make it easier to discern between commenting and adding a new answers to a question.

Answers rich text editor update - The rich text editor has been updated to the 19b version of the MATLAB Live Editor. This update will primarily help with syntax highlighting along with resolving a few other issues.
Answer pages translation option - Answers translations options added. A translation option for questions and answers content has been added for French, German, Italian, Spanish, and Chinese languages.

Hey everyone! I'm spotlighting Nikolaos Nikolaou today because of the sheer quantity of his Cody solutions over such a relatively short time span. Nikolaos has submitted 1,161 Cody solutions over the last 6 months of 2019, averaging 6.4 solutions per day. Achieving a Cody rank of 19 with a score of 18,820 and earning him a plethora of badges (including the Speed Demon badge of course). He's completed 24 problem groups and is well into most of the remaining 35.
Way to go Nikolaos!
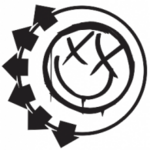
Check out nextname by Stephen Cobeldick, this weeks pick by Jiro. Read Jiro's blog post about why he picked Stephen's submission this week.

Please post any comments to Jiro's blog post here.
I'm please to announce this new MATLAB Central feature for posting community highlights. This new channel will:
- Allow MathWorks and the community leaders to easily post newsworthy items to the community
- Allow community visitors to respond to these posts with Likes and Replies
- Allow anyone to follow/subscribe the channel so they can be notified of new posts
What do we mean by newsworthy? In short, this means anything we think some or all of the community might like to know. Here are some examples of what we’re thinking about posting to this new channel.
- New or upcoming community features or events
- User highlights (e.g. examples of good behavior, interesting posts)
- Interesting content (e.g. File Exchange pick of the week submissions)
- Release notes and new features
- Polls (future)
New highlights will appear on the community home page at the time they are posted and all past highlights can be found by going to the community home page and clicking the Highlights link in the right column.
As always, let us know what you think by liking this post or commenting below.

The following is a list of updates and new features for MATLAB Central, including MATLAB Answers, File Exchange, Blogs, and Cody.
New Features
Profile search - A global community profile search has been added. The search field on community profile pages has been updated from a standard content search to a user profile search. This improvement makes it easier to find community members across all MATLAB Central. Previously one had to search the Answers contributors , File Exchange authors , and Cody players page when looking for a user profile.

Last seen - We have added a 'last seen' timestamp to community profiles which displays the date of a person's last visit to MATLAB Central. This can be a relevant bit of information and help determine how recent someone has been active in the community.
Answers pages design update - Answers Q&A pages have been updated to remove extra white space. This update includes smaller sized avatars, and position changes for the voting and content actions among other small changes. All these changes also help improve the mobile experience as well.
Original poster styles - Original poster styles have been introduced in Answers. When a question author participates in a Q&A thread their comments or answers will be styled with a blue background and left border so they're easily discernable from other contributors.

File Exchange data in monthly emails - File Exchange stats will be included in the monthly email we send to contributors who've participated in the community on any given month.
Trending content algorithm - The MATLAB Central home page trending content algorithm has been updated to look at content activity over a shorter period of time resulting in a more dynamic feed.
Walter Roberson does it again by winning the coveted MOST ACCEPTED answers badge for all his contributions in MATLAB Answers this past year. Walter has won this badge every year since 2015. It was way back in 2014 when Image Analyst out paced Walter and was awarded the badge.
There are 10 community members who have achieved the Top Downloads badge for their popular File Exchange submissions in 2019. Do you recognize any of these names? There's a good chance you've used one or more of their toolboxes or scripts in your work if you're a frequent visitor to File Exchange, if you're not you might want to check out what they've posted, it may save you a lot of time writing your own code.
--------------------- Top Downloads Badge Winners -----------------
- Diego Barragán
- Dirk-Jan Kroon
- Yi Cao
- John D'Errico
- Yair Altman
- Giampiero Campa
- Michael Kleder
- Dr. Siva Malla
- Antonio Trujillo-Ortiz
- Brett Shoelson
Congratulations to all these winners and a giant THANK YOU for all they've done this past year to help everyone in the MATLAB Central community!

We are happy to announce that virtual badges can now be achieved for participating in MATLAB Central File Exchange . We have 30 badges that anyone can achieve, which will also boost your community profile. Some badges are relatively simple to get while others will depend on how useful your submissions are to others in the community. Check out Ned Gulley's blog post for a great introduction.

Explore resources, ask questions, and discuss topics related to using Simulink to apply power electronics control to Electric Vehicles, Renewable Energy, Battery Systems, Power Conversion, and Motor Control. This is the 3rd MATLAB Central community, after Maker and SimBiology , and is moderated by Tony Lennon . Tony is the Power Electronics Marketing Manager at MathWorks.
Visit the community here . As always, let us know what you think by liking this post or commenting below.
